How to make a UNIX/Linux 'daemon'
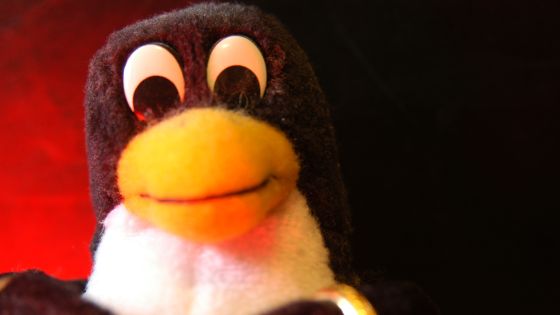
by Jeff Hitchcock
When we look into ways to make Windows and Android devices work better, we see words such as 'task', 'process', and 'daemon'. Especially in UNIX/Linux, the daemon plays the role of a web server or mail server as a background process that does not have a control terminal for interacting with users. Engineer Aryaman Sharda explains how to create that daemon.
Understanding Daemons (in Unix)-Digital Bunker
https://blog.digitalbunker.dev/2020/09/03/understanding-daemons-unix/
A daemon is a process that runs in the background and is waiting for a specific event to occur. Typical daemons are httpd of web server and sshd which provides SSH connection.
Sharda says that UNIX/Linux starts a process called init when the OS boots and assigns '1' to init as the PID, which is the process identification number. init is a daemon with no controlling terminal, and all processes are child processes started by init. If you run the ' pstree ' command on Linux, you can see that init is at the top of every process.
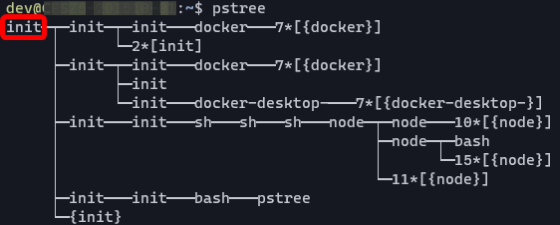
When booting is completed, the OS will start the prepared daemon. These daemons are created by the fork function that creates a child process and the exit function that ends the process normally, and eventually becomes a process that has the init process as its parent process.
Also, modern Linux distributions use systemd , so the process with PID 1 which is the parent process of all processes is generally systemd.
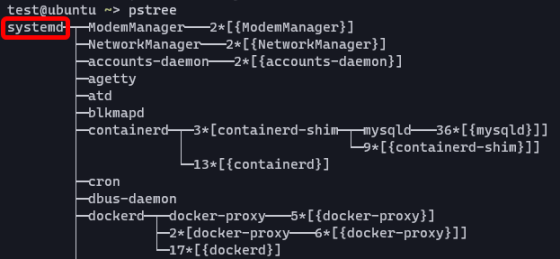
To create a new daemon, first call the fork function to create a child process.
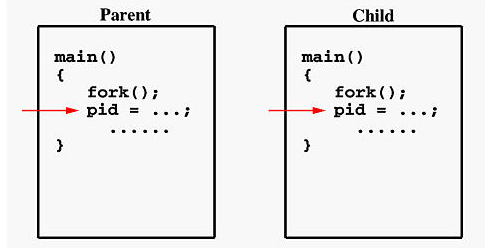
The child process shares the CPU register of the parent process, so both the generated child process and the parent process execute the instructions after the fork function in parallel, Sharda explained. If you exit the parent process with the exit function, the child process will automatically become a daemon with init as the parent process.
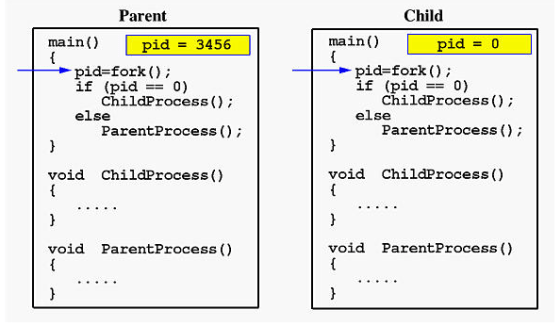
When actually implementing the daemon, a little more complicated processing is required. Sharda explains how to create a daemon based on the sample code below.
The first process in the create_daemon function to create a daemon is to initialize the variable pid and call the fork function to create a child process.
[code]
static void create_daemon()
{
pid_t pid;
pid = fork();
[/code]
Also check if the fork function has finished properly. If the PID is a positive value, it is determined that the child process was created correctly.
[code]
if (pid <0)
exit(EXIT_FAILURE);
if (pid> 0)
exit(EXIT_SUCCESS);[/code]
Then create a new session with the setsid function. A session is a collection of processes corresponding to one controlling terminal, and when the parent process that is the session leader ends, all child processes also end. However, when you create a new session, the session does not have a controlling terminal. It seems that the daemon creation was completed by being able to create a process without a controlling terminal, but processing continues.
..
[code]
if (setsid() <0)
exit(EXIT_FAILURE);[/code]
Signals sent from the control terminal and child processes are ignored, and processing is performed so that daemon generation is not affected.
[code]
signal(SIGCHLD, SIG_IGN);
signal(SIGHUP, SIG_IGN);[/code]
Call the fork function again after creating a new session. Although the setsid function is used to create a process that does not have a controlling terminal, as it is, the process is the session leader of a new session, so if the user opens another terminal, the controlling terminal may be acquired. There is. To ensure that a daemon with no controlling terminal is created, first move the process to a new session different from the existing session with the setsid function and then create a child process again to prevent association with the controlling terminal. By terminating the parent process, the chance of getting the controlling terminal is zero.
[code]
pid = fork();
if (pid <0)
exit(EXIT_FAILURE);
if (pid> 0)
exit(EXIT_SUCCESS);[/code]
Next, set the umask when creating a new file or directory. In UNIX/Linux, the permission when creating a new file is 0666 (owner, group, and others can read and write), and the directory is created with 0777 with execution permission added to 0666, but if umask is set to 0022, for example, File is created with 0644 (owner can read/write, group, others can only read), directory has 0755 (owner can read/execute, group, others can only read/execute) Will be. This code sets umask to 0000.
[code]umask(0);[/code]
Go to the root directory and close all file descriptors the process has.
[code]
chdir('/');
int x;
for (x = sysconf(_SC_OPEN_MAX); x>=0; x--)
{
close (x);
}[/code]
After that, call the create_daemon function in the main function and describe the process in the infinite loop to complete the daemon.
[code]
int main()
{
create_daemon();
while (1)
{
Some kind of processing
}
return EXIT_SUCCESS;
}[/code]
By the way, the word
What is the origin of the 'Daemon' process often used in UNIX/Linux? -GIGAZINE
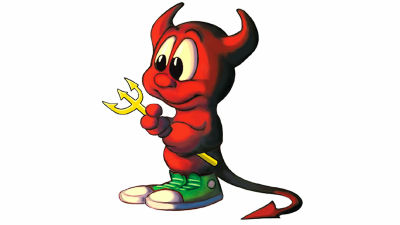
Related Posts:
in Software, Posted by darkhorse_log