What does Linus Torvalds, the creator of Linux, think of as 'good code'?
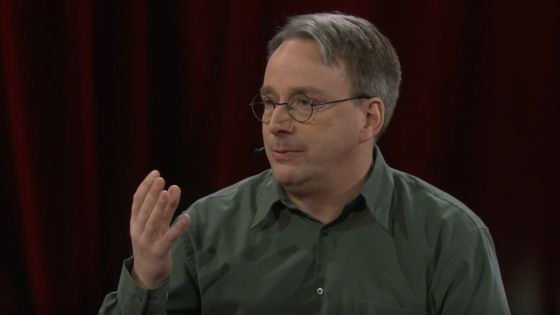
When programming, it is important to aim for ``easy-to-read code'' by leaving comments properly and giving easy-to-understand variable names. However, it is also true that there is sometimes a big structural difference between 'readable code' and 'good code'. Engineer
mkirchner/linked-list-good-taste: Linus Torvalds' linked list argument for good taste, explained
https://github.com/mkirchner/linked-list-good-taste
Linus Torvalds: The mind behind Linux | TED Talk
https://www.ted.com/talks/linus_torvalds_the_mind_behind_linux?language=ja
In a 2016 TED interview , Torvalds used ``how to implement linked lists'' as an example to explain his thoughts on ``good code.'' A linked list is a list that connects data linearly, and is one of the structures for handling data. Specifically, a linked list is a series of 'boxes' consisting of values such as '4' or '12' and a pointer indicating the 'next value.'

The code that implements the linked list in C language is below. An 'IntListItem' structure is created with 'value' which is the value itself and 'next' which is a pointer indicating the next value, and a box is generated from the IntListItem structure. The 'IntList' structure has a variable 'head' that represents the beginning of the list.
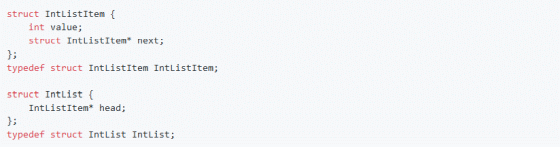
The code introduced in Stanford University's computer science lecture '
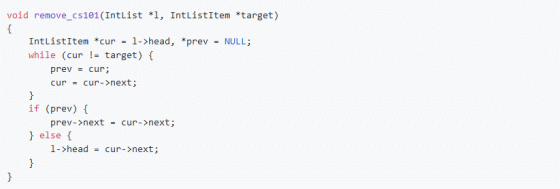
The process performed by the above code is as follows. First, use the following part of the code to search for the 'box you want to erase' from the top of the list. Since a linked list can only trace values from the beginning of the list, first store the address of the box at the beginning of the list in the 'cur' pointer, and initialize the 'prev' pointer with NULL. Match by moving the cur pointer to the address of the next box until the cur pointer matches the address of the box you want to erase.
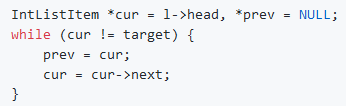
Below is a diagram showing the image of the above process. It looks like the prev pointer and cur pointer are sliding into the box at the end of the list.
Once the cur pointer matches the address of the box to be erased, the next step is to erase the box. In the process below, if the box to be erased is not the first in the list, the address of the box following the box to be erased is stored in the next pointer of the box before the box to be erased. If the box to be erased is the first in the list, store the address of the next box after the box to be erased in the head pointer pointing to the beginning of the list.
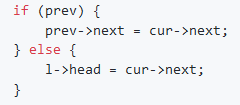
A diagram explaining the process when the box to be deleted is not at the beginning of the list looks like this. The next pointer of the previous box to be deleted will now point to the next box to be deleted, so the box to be deleted will no longer be referenced by any box and will be deleted from the list. In the image below, the box with the value '12' has been cleared.
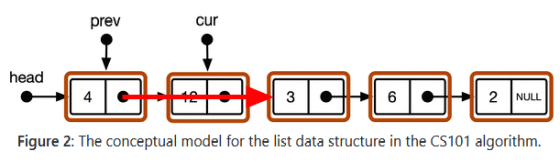
If the box to be erased is the first box in the list, process the head pointer to point to the address of the second box from the list as shown below.
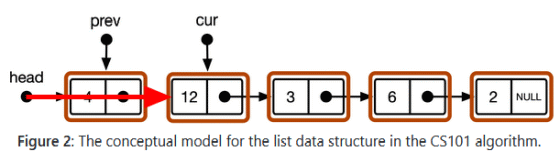
It is certainly possible to delete elements from a list using the code introduced in CS101. However, Torvalds introduced an even better code in a 2016
The code by Torvalds is below. The if statement that was in the previous code has disappeared, making it a simpler code.
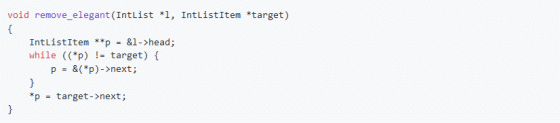
The major difference between C101's code and Mr. Torvald's code is that a new pointer '**p' called 'pointer of box pointer' is introduced. First, '**p' is initialized as a 'head pointer pointer'.

If the box pointer '*p' does not match the address of the box to be erased, change it to 'pointer of next box pointer'.
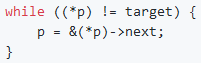
The diagram looks like this, and the 'box pointer pointer' moves to the box at the back of the list.
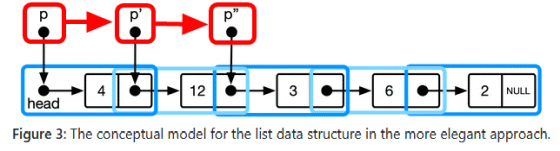
When the box to be erased is found, the while statement is exited and the box pointer is moved to the address of the next box to be erased, thereby removing the box from the list.

The deletion process looks like this.
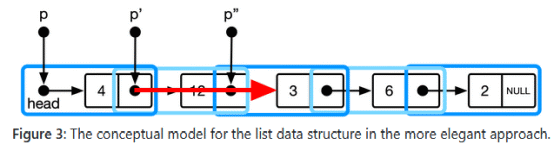
The improved code by Torvalds eliminates the need for conditional branching based on the state of the head pointer by introducing a 'pointer of pointers.' Additionally, there is no need to substitute two variables, cur and prev, simplifying the process.
'I want people to understand that sometimes you can look at a problem from a different angle and rewrite the code by reducing the special case to the normal case, and that's what makes good code,' Torvalds said in a TED interview. That's it,' he commented. Mr. mkirchner also implemented code to add a box based on the code by Mr. Torvalds, and published it on GitHub.
Related Posts:
in Software, , Posted by darkhorse_log