A library ``GPU.js'' that can easily handle GPU with JavaScript is reviewed, multidimensional operation is explosive with parallel processing
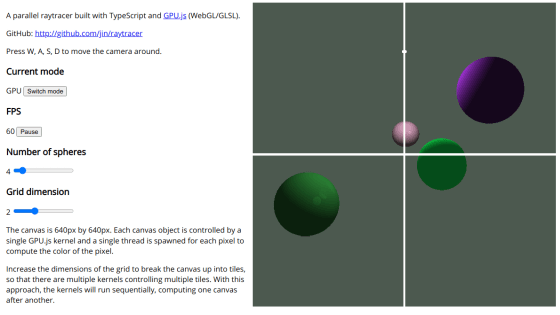
Speaking of a programming language used for parallel computing using a GPU, Python is commonly used for research on machine learning, but you may want to use the GPU in a JavaScript web application. By using the open source JavaScript library ' GPU.js ', you can perform multi-dimensional calculations at high speed by performing parallel processing using the GPU of the computer that executes the script.
GPU.js-GPU accelerated JavaScript
The system that runs GPU.js this time is as follows. CPU uses Intel Core i5-4570, GPU uses AMD Radeon RX480, OS uses Ubuntu 20.04, and window system uses Wayland .
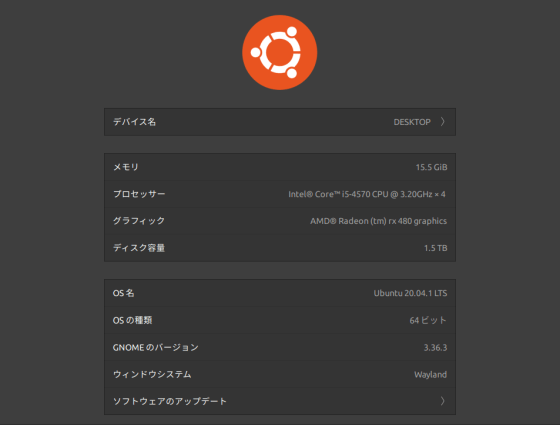
Kernel version is 5.4.0-42

The driver loading is like this. It uses the driver that comes standard with Linux.
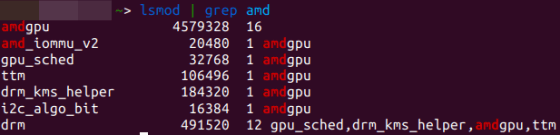
In order to execute JavaScript using GPU.js in Node.js, install the package with the following command. The version of Node.js used this time is 12.8.2.
[code]npm install gpu.js --save[/code]
How to use GPU.js in Node.js is like this. First, load gpu.js with require.
[code]const {GPU} = require('gpu.js');[/code]
Then initialize the 'GPU' object and create a 'gpu' instance.
[code]const gpu = new GPU();[/code]
In the method 'createKernel' of the created gpu instance, describe the calculation you want to execute on the GPU.
[code]const multiplyMatrix = gpu.createKernel(function(a, b) {
let sum = 0;
for (let i = 0; i <1024; i++) {
sum += a[this.thread.y][i] * b[i][this.thread.x];
}[/code]
First, as a comparison target with GPU, I will execute JavaScript including two-dimensional matrix operation only on the CPU. If you specify '{ mode:'cpu', }' when creating an instance, you can calculate using CPU.
[code]const generateMatrices = () => {
const matrices = [[], []]
for (let y = 0; y <1024; y++){
matrices[0].push([])
matrices[1].push([])
for (let x = 0; x <1024; x++){
matrices[0][y].push(Math.random())
matrices[1][y].push(Math.random())
}
}
return matrices
}
const {GPU} = require('gpu.js');
const gpu = new GPU({ mode:'cpu', });
const multiplyMatrix = gpu.createKernel(function(a, b) {
let sum = 0;
for (let i = 0; i <1024; i++) {
sum += a[this.thread.y][i] * b[i][this.thread.x];
}
return sum;
}).setOutput([1024, 1024])
const matrices = generateMatrices()
const out = multiplyMatrix(matrices[0], matrices[1])
console.log(out[10][12])[/code]
The result of checking the
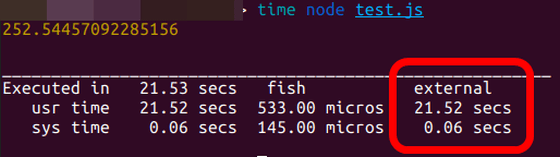
Next, let's try using the GPU. Remove the option to create a 'GPU' instance and try running the script again.
[code]const gpu = new GPU();[/code]
The execution result is as follows. You can see that the execution time is 555.28 milliseconds (about 0.56 seconds), which is much faster than when it was executed on the CPU.
Next, let's verify the speed at which a normal image is displayed. First, use the following HTML to measure the display speed when GPU.js is not used.
[code]GPU.js test
[/code]
The execution result looks something like this.
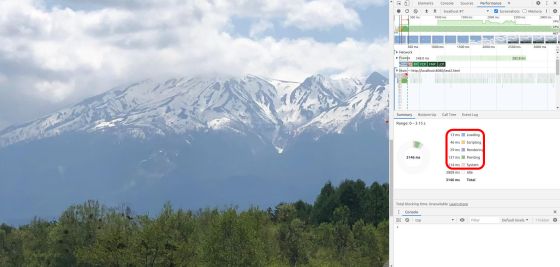
Let's check the display speed when an image is displayed using the processing by GPU.js.
[code]
GPU.js test
[/code]
Compared to the case where GPU.js is not used, the number of seconds other than 'idle' has increased. Obviously, it doesn't seem to be suitable for applications that do not require parallel processing, such as simply displaying an image.
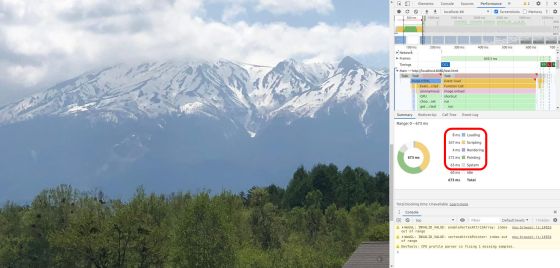
In addition, GPU.js also provides a wealth of samples to help you implement it. For example, when you display 'slow-fade.html' where the color changes slowly, it looks like this.
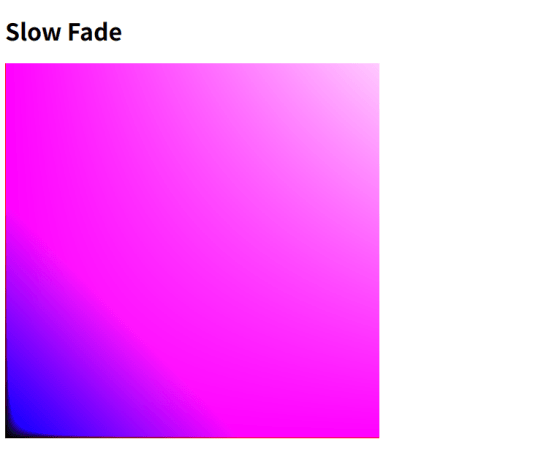
In GPU mode,
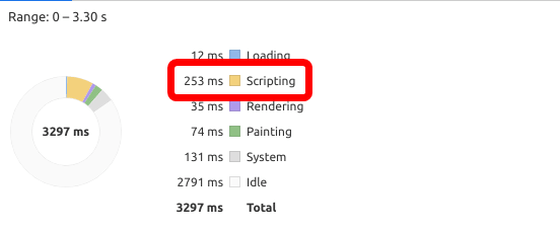
When I switched to CPU mode, it took 1700 milliseconds. You can see the power of parallel computing with GPU.
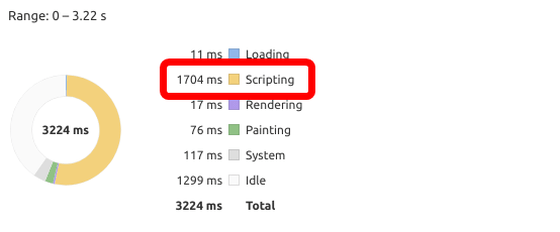
In addition, 'parallel-raytracer.html' that implements ray tracing is prepared as a sample.
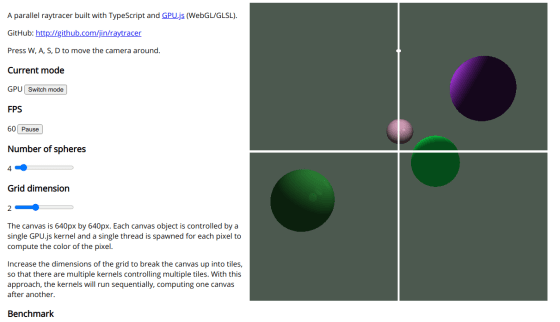
There is also a benchmark site that allows you to easily measure the effects of using GPU.js.
GPU.js-GPU accelerated JavaScript
Access the browser by URL and click 'BENCH MARK!'.
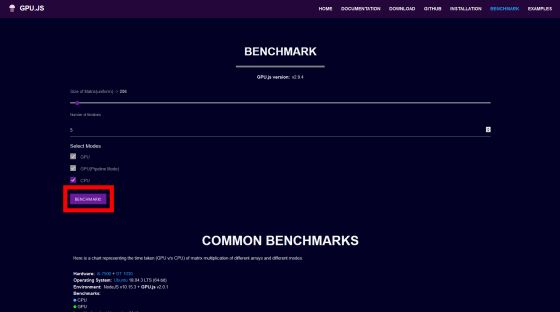
Benchmark results are displayed. The top is the score when using GPU, and the bottom is the score when using CPU.
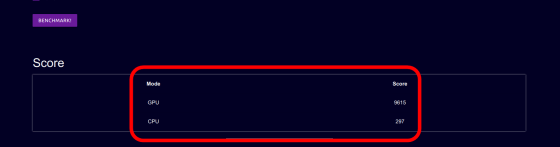
The measurement results of a notebook PC without an external GPU equipped with Intel Core i7-6500U are as follows. You can see that the processing is faster even with the built-in GPU.
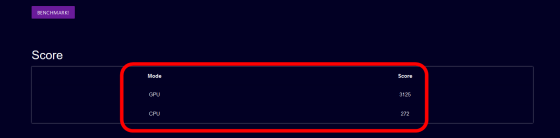
Since Pixel 3 also has Adreno 630 as a GPU, measuring the benchmark shows that the processing speed is still better when using the GPU than when only using the CPU. Access from devices that do not support GPU will automatically fall back to calculation by the CPU, so it is possible to avoid the situation where some GPU.js embedded pages are not displayed on some devices. When performing calculations such as multidimensional vectors in JavaScript, it seems that performance improvement can be expected by incorporating GPU.js.
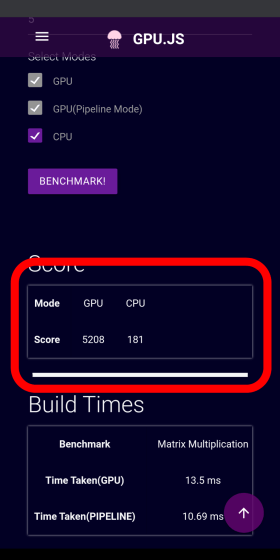
The source code of GPU.js is published on GitHub, and anyone can use it.
GitHub-gpujs/gpu.js: GPU Accelerated JavaScript
https://github.com/gpujs/gpu.js/#gpucreatekernel-settings
Related Posts: